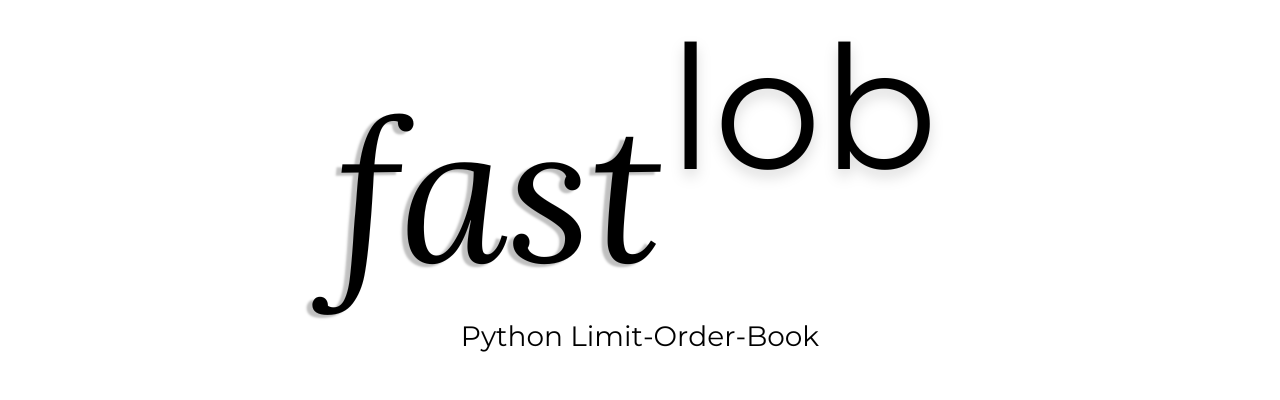
Fast & minimalist limit-order-book implementation in pure Python. This package can be used for both live order processing and simulating historical high-frequency market data.
This package is still being developed, bugs are expected. Do not use in production.
Quickstart
To install the package you can either install it using pip:
pip install fastlob
Otherwise, you can build the project from source:
git clone git@github.com:mrochk/fastlob.git
cd fastlob
pip install -r requirements.txt
pip install .
Examples
Placing a limit GTD order and getting his status.ΒΆ
1import time, logging
2from fastlob import Orderbook, OrderParams, OrderSide, OrderType
3
4logging.basicConfig(level=logging.INFO) # set maximum logging level
5
6lob = Orderbook(name='ABCD', start=True) # create a lob an start it
7
8# create an order
9params = OrderParams(
10 side=OrderSide.BID,
11 price=123.32,
12 quantity=3.4,
13 otype=OrderType.GTD,
14 expiry=time.time() + 120 # order will expire in two minutes
15)
16
17result = lob(params); assert result.success() # place order
18
19status, qty_left = lob.get_status(result.orderid()) # query status of order
20print(f'Current order status: {status.name}, quantity left: {qty_left}.\n')
21
22lob.render() # pretty-print the lob
23
24lob.stop() # stop background processes